Use this Python code to automatically mint XENFTs at the lowest possible gwei, saving you money on Ethereum transaction fees.
XENFTs are non-fungible tokens that can be bought and sold on OpenSea, and minted on the Ethereum network. They are part of the XEN Crypto project, which aims to promote the use and adoption of XEN as a medium of exchange. XEN Torrent (creation mechanism for XENFTs) is implemented as an ERC-721 compatible smart contract that adheres to the principles of decentralization, including no pre-minting and no special allocations. They can be used in conjunction with XEN’s Proof of Burn protocol (APEX and LIMITED category) and can be deployed on any EVM-compatible network where the XEN Crypto contract is operational (at the moment only on the Ethereum blockchain).
However, the minting of a XENFT requires paying for the Ethereum transaction fees, which can add up quickly, especially if you’re planning to create a XENFT with over 100 VMUs. The following Python code allows you to mint XENFTs at the lowest possible gwei, saving you money on Ethereum transaction fees.
Important: As of this moment, the below Python code can only be used for minting the 8 different Collector XENFT categories. Support for APEX and LIMITED XENFTs is not built in, but feel free to fork the GitHub repository and add the support.
VIDEO DEMO
https://www.youtube.com/watch?v=XAfgy-RgMcA
Prerequisites
To run and execute the below code, you’ll need Python 3.9 (we’ve tested on this version) installed on your Windows or Linux system. You will need to make sure you have the following prerequisites installed:
- Python 3.9: You can download and install the latest version of Python from the official website (https://www.python.org/downloads/). Make sure to choose the correct version for your operating system (Windows or Linux).
- A text editor: You will need a text editor to write your Python code. Some popular options include Sublime Text, Visual Studio Code or PyCharm.
- A terminal or command prompt: You will need a terminal or command prompt to run your Python code. On Windows, you can use the Command Prompt or PowerShell. On Linux, you can use the default terminal application.
- Basic knowledge of Python: It is recommended that you have some basic knowledge of Python before running and executing code. This includes understanding concepts such as variables, loops, and functions.
- Once you have these prerequisites installed and set up, you should be ready to run and execute the below XENFT Minter (Python 3.9 code) on your Windows or Linux system.
Disclaimer
You are solely responsible for ensuring the secure configuration and operation of this script, including but not limited to protecting it from unauthorized access by third parties. Failure to do so may result in the loss of your private keys, XENFTs or other cryptocurrencies stored in your account.
You should exercise caution and only run this script on a secure workstation or server. By using this script, you acknowledge that you understand the inherent risks associated with running this script and that you agree to use this script at your own risk.
The creator of this script will not be held liable for any damages, loss of funds, or other negative consequences that may result from you or any third party using this script.
You are solely responsible for any actions taken using this script, and for securing your private keys and other sensitive information. This script is provided ‘as is,’ and the creator makes no warranty, express or implied, of any kind.
How to Configure the script
To use this code, you’ll need to enter a few configuration details that pertain to your wallet.
One of them is your wallet address (also called a public key) which you can hardcode into the script.
The second one is your private key. This one is not recommended to save into the script itself, rather we’ve created a prompt that will ask you for this information at runtime (that way your private key is not stored in the script itself).
your_wallet_address = '0x' # replace with the account from which we'll pay for XENFT (ensure you have sufficient funds)
your_wallet_address_private_key = getpass.getpass(prompt="Please enter your wallet private key: ")
# NOT SECURE & NOT RECOMMENDED: Uncomment the following line to hardcode your wallet's private key
your_wallet_address_private_key = '012345abcdef...'
The number of VMUs (virtual machine units) that you want to mint:
vmu = 128 # How many VMUs to mint
You can also set the Maximum Term for your XENFTs, which determines how long it’ll take until you can claim them (or you can use the automated function to determine the current maximum term):
Manual Max Term:
manual_max_term = 440 # Hardcoded max term for your XENFT
In addition to minting XENFTs, this code also includes a function to fetch the current maximum term for XENFTs, which is useful if you want to keep track of how long your XENFTs can be claimed for.
Automatic Max Term:
if TRUE script will automatically return max term and overwrite above manual_max_term value.
If FALSE, script will default to manually configured value (manual_max_term)
use_automatic_max_term = True
Gas-related configuration includes the ability to set the maximum price at which you want to execute the XENFT mint. For example, if only_claim_if_gas_is_below = 15, the script will wait for the Ethereum gas fees to drop to 15 gwei. You can set up max priority per gas as well, but leaving it at 1 is a good default value.
#Set gas-related claiming parameters
only_claim_if_gas_is_below = 15
max_priority_fee_per_gas = 1
Claim consecutive parameters are important to ensure that gas did not drop just for one second before we go ahead and claim XENFT, as that could cause your XENFT to be stuck. Here you can configure the number of consecutive times that gas fell below the value you configured earlier. For example, if claim_when_consecutive_count = 3, and how_many_seconds_between_checks = 10, the script will only go ahead and mint XENFT if 3 consecutive gas price checks fell below the value you’ve provided. Each of those checks will take place in 10-second intervals.
# Claim/Mint parameters
claim_when_consecutive_count = 3 # if n checks in a row are at below OnlyClaimIfGasBelow, only then claim XENFT
how_many_seconds_between_checks = 10
Infura URL – All requests to Entrust network must go through the Ethereum RPC node. If you want to use Infura nodes, you must have a valid API key appended to Infura request URL. You can get your own Infura API key at: https://infura.io. If you don’t know how to go about it, watch the following video guide: https://youtu.be/R2WkpF4Em7k). Once you have the key, adjust the URL below accordingly:
rpc_url = "https://mainnet.infura.io/v3/ABCDEF # Replace ABCDEF with your Infura API id
Alternatively, if you don’t want to sign up with Infura and monitor your own requests, just use a Public Ethereum RPC, such as this one (below) or get one from: https://llamanodes.com/public-rpc
rpc_url = "https://eth.llamarpc.com"
How to Mint XENFT using the Python script
Once you’ve entered the necessary configuration details, the code will automatically check the current gas price and only mint XENFTs if the gas price is below a certain threshold for a number of consecutive times you’ve specified. This way, you should be able to ensure that you’re paying the lowest possible price that you’re willing to pay for your XENFTs.
In order to execute the script, save it into YOUR_CODE_DIRECTORY under the name: xenft-creator.py
On Windows, you can execute the script by running it like this:
C:\YOUR_PYTHON_INSTALL_DIRECTORY\python.exe C:\YOUR_CODE_DIRECTORY\xenft-creator.py
On Linux:
/usr/bin/python3 /home/xenft-creator.py
Once the script running, it should look something like this, and you’ll see that the script is attempting to mint XENFT:
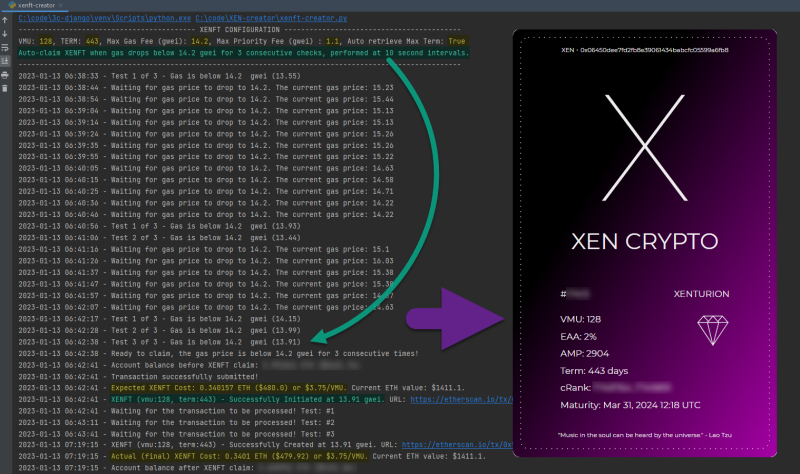
Once the XENFT is minted, the script will provide:
- URL to Etherscan
- Your wallet’s account balance before and after the XENFT mint
- The overall XENFT cost in Ethereum as well as in US dollars
- The average Cost per VMU in Ethereum as well as in US dollars
-
Conclusion
Overall, this Python code should be a useful tool for anyone looking to mint XENFTs at the lowest possible gwei. Whether you’re an experienced Ethereum user or new to the world of cryptocurrency, this code is easy to use and can help you save money on Ethereum transaction fees. Configure it with a reasonable gwei value, then start the python script and let it run overnight. By the time you wake up, your XENFT should be minted and waiting for you 🙂
Enjoy and leave a donation if this code helped you to save some money.
Open Source Code
The full script, released as an open source under the MIT license is here:
https://github.com/JozefJarosciak/xenft-creator