How high the max term goes?
The following XEN Max Term Calculator visualizes the Global Rank’s impact on XEN Max Mint Term.
As of today (15 Oct 2023), we have a Global Rank of 18,471,672 accounts minting #XEN on #Ethereum. That results in the Max Term of 462 days. Now, how about the theoretical maximum? Well, based on the calculation at 100 Billion accounts (meaning, if every person on earth had 12 wallets minting XEN), we would see a max term of 648 days. Not that that will ever happen, but that's in my opinion how high it can go on some smaller chains, where single minters can easily mint tens of thousands of accounts.
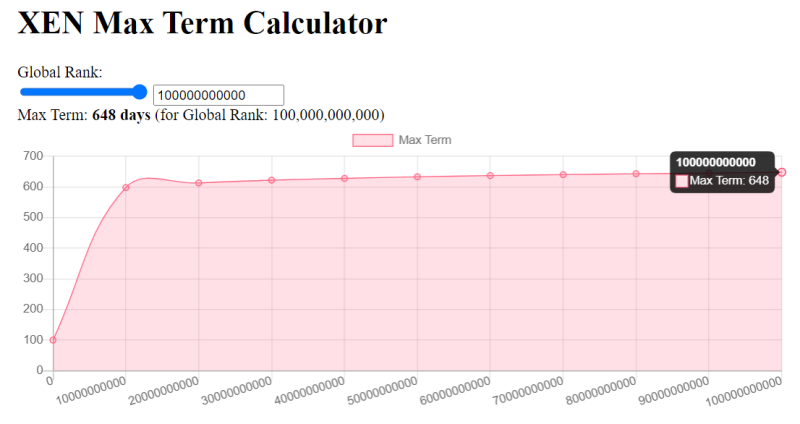
Code explanation
This is the full code of the entire script that calculates XEN’s maximum term for a given Global Rank. It uses functions to calculate the maximum term (created by converting XEN solidity function to JavaScrit), the Chart.js library to generate the graph and a function to format the output numbers. It also contains HTML to allow users to input Global Rank through input box as well as by using the dynamic slider:
For reference, here is the XEN smart contract solidity function that XEN contract uses to calculate the max term:
uint256 public constant SECONDS_IN_DAY = 3_600 * 24;
uint256 public constant MAX_TERM_START = 100 * SECONDS_IN_DAY;
uint256 public constant MAX_TERM_END = 1_000 * SECONDS_IN_DAY;
uint256 public constant TERM_AMPLIFIER = 15;
uint256 public constant TERM_AMPLIFIER_THRESHOLD = 5_000;
function _calculateMaxTerm() private view returns (uint256) {
if (globalRank > TERM_AMPLIFIER_THRESHOLD) {
uint256 delta = globalRank.fromUInt().log_2().mul(TERM_AMPLIFIER.fromUInt()).toUInt();
uint256 newMax = MAX_TERM_START + delta * SECONDS_IN_DAY;
return Math.min(newMax, MAX_TERM_END);
}
return MAX_TERM_START;
}
The above Solidity function calculates the maximum mint term (in seconds) for of a particular Global Rank. It first checks if the Global Rank is above the TERM_AMPLIFIER_THRESHOLD. If so, it calculates the delta by taking the logarithm of the global rank and multiplying it by the TERM_AMPLIFIER. It then calculates the new maximum term start by adding the delta multiplied by SECONDS_IN_DAY (which is the number of seconds in a day) to the MAX_TERM_START. It then returns either the new maximum term start or the MAX_TERM_END whichever is smaller.
I’ve taken that function and converted it to JavaScript (code example below). The JavaScript function works exactly the same way as the Solidity function, with one exception, and that is, instead of the max mint term being returned in seconds, it returns the result converted to a number of days.
Open-source & interactive graph: https://jsfiddle.net/jarosciak/c5abt7m6/
(feel free to make it better)
I hope this explains how the maximum day is calculated.
Enjoy!